line |
stmt |
bran |
cond |
sub |
pod |
time |
code |
1
|
|
|
|
|
|
|
package Chart::Plotly::Trace::Funnel; |
2
|
1
|
|
|
1
|
|
797
|
use Moose; |
|
1
|
|
|
|
|
3
|
|
|
1
|
|
|
|
|
9
|
|
3
|
1
|
|
|
1
|
|
7637
|
use MooseX::ExtraArgs; |
|
1
|
|
|
|
|
3
|
|
|
1
|
|
|
|
|
12
|
|
4
|
1
|
|
|
1
|
|
2496
|
use Moose::Util::TypeConstraints qw(enum union); |
|
1
|
|
|
|
|
3
|
|
|
1
|
|
|
|
|
12
|
|
5
|
|
|
|
|
|
|
if ( !defined Moose::Util::TypeConstraints::find_type_constraint('PDL') ) { |
6
|
|
|
|
|
|
|
Moose::Util::TypeConstraints::type('PDL'); |
7
|
|
|
|
|
|
|
} |
8
|
|
|
|
|
|
|
|
9
|
1
|
|
|
1
|
|
1318
|
use Chart::Plotly::Trace::Funnel::Connector; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
48
|
|
10
|
1
|
|
|
1
|
|
706
|
use Chart::Plotly::Trace::Funnel::Hoverlabel; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
53
|
|
11
|
1
|
|
|
1
|
|
716
|
use Chart::Plotly::Trace::Funnel::Insidetextfont; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
49
|
|
12
|
1
|
|
|
1
|
|
698
|
use Chart::Plotly::Trace::Funnel::Marker; |
|
1
|
|
|
|
|
5
|
|
|
1
|
|
|
|
|
44
|
|
13
|
1
|
|
|
1
|
|
597
|
use Chart::Plotly::Trace::Funnel::Outsidetextfont; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
46
|
|
14
|
1
|
|
|
1
|
|
595
|
use Chart::Plotly::Trace::Funnel::Stream; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
40
|
|
15
|
1
|
|
|
1
|
|
591
|
use Chart::Plotly::Trace::Funnel::Textfont; |
|
1
|
|
|
|
|
6
|
|
|
1
|
|
|
|
|
45
|
|
16
|
1
|
|
|
1
|
|
643
|
use Chart::Plotly::Trace::Funnel::Transform; |
|
1
|
|
|
|
|
4
|
|
|
1
|
|
|
|
|
1077
|
|
17
|
|
|
|
|
|
|
|
18
|
|
|
|
|
|
|
our $VERSION = '0.041'; # VERSION |
19
|
|
|
|
|
|
|
|
20
|
|
|
|
|
|
|
# ABSTRACT: Visualize stages in a process using length-encoded bars. This trace can be used to show data in either a part-to-whole representation wherein each item appears in a single stage, or in a "drop-off" representation wherein each item appears in each stage it traversed. See also the "funnelarea" trace type for a different approach to visualizing funnel data. |
21
|
|
|
|
|
|
|
|
22
|
|
|
|
|
|
|
sub TO_JSON { |
23
|
1
|
|
|
1
|
1
|
3
|
my $self = shift; |
24
|
1
|
|
50
|
|
|
38
|
my $extra_args = $self->extra_args // {}; |
25
|
1
|
|
|
|
|
12
|
my $meta = $self->meta; |
26
|
1
|
|
|
|
|
23
|
my %hash = %$self; |
27
|
1
|
|
|
|
|
5
|
for my $name ( sort keys %hash ) { |
28
|
4
|
|
|
|
|
180
|
my $attr = $meta->get_attribute($name); |
29
|
4
|
100
|
|
|
|
28
|
if ( defined $attr ) { |
30
|
3
|
|
|
|
|
5
|
my $value = $hash{$name}; |
31
|
3
|
|
|
|
|
80
|
my $type = $attr->type_constraint; |
32
|
3
|
50
|
33
|
|
|
20
|
if ( $type && $type->equals('Bool') ) { |
33
|
0
|
0
|
|
|
|
0
|
$hash{$name} = $value ? \1 : \0; |
34
|
|
|
|
|
|
|
} |
35
|
|
|
|
|
|
|
} |
36
|
|
|
|
|
|
|
} |
37
|
1
|
|
|
|
|
82
|
my $plotly_meta = delete $hash{'pmeta'}; |
38
|
1
|
50
|
|
|
|
5
|
if ( defined $plotly_meta ) { |
39
|
1
|
|
|
|
|
5
|
$hash{'meta'} = $plotly_meta; |
40
|
|
|
|
|
|
|
} |
41
|
1
|
|
|
|
|
5
|
%hash = ( %hash, %$extra_args ); |
42
|
1
|
|
|
|
|
3
|
delete $hash{'extra_args'}; |
43
|
1
|
50
|
33
|
|
|
11
|
if ( $self->can('type') && ( !defined $hash{'type'} ) ) { |
44
|
1
|
|
|
|
|
4
|
$hash{type} = $self->type(); |
45
|
|
|
|
|
|
|
} |
46
|
1
|
|
|
|
|
4
|
return \%hash; |
47
|
|
|
|
|
|
|
} |
48
|
|
|
|
|
|
|
|
49
|
|
|
|
|
|
|
sub type { |
50
|
1
|
|
|
1
|
1
|
5
|
my @components = split( /::/, __PACKAGE__ ); |
51
|
1
|
|
|
|
|
4
|
return lc( $components[-1] ); |
52
|
|
|
|
|
|
|
} |
53
|
|
|
|
|
|
|
|
54
|
|
|
|
|
|
|
has alignmentgroup => ( |
55
|
|
|
|
|
|
|
is => "rw", |
56
|
|
|
|
|
|
|
isa => "Str", |
57
|
|
|
|
|
|
|
documentation => |
58
|
|
|
|
|
|
|
"Set several traces linked to the same position axis or matching axes to the same alignmentgroup. This controls whether bars compute their positional range dependently or independently.", |
59
|
|
|
|
|
|
|
); |
60
|
|
|
|
|
|
|
|
61
|
|
|
|
|
|
|
has cliponaxis => ( |
62
|
|
|
|
|
|
|
is => "rw", |
63
|
|
|
|
|
|
|
isa => "Bool", |
64
|
|
|
|
|
|
|
documentation => |
65
|
|
|
|
|
|
|
"Determines whether the text nodes are clipped about the subplot axes. To show the text nodes above axis lines and tick labels, make sure to set `xaxis.layer` and `yaxis.layer` to *below traces*.", |
66
|
|
|
|
|
|
|
); |
67
|
|
|
|
|
|
|
|
68
|
|
|
|
|
|
|
has connector => ( is => "rw", |
69
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Connector", ); |
70
|
|
|
|
|
|
|
|
71
|
|
|
|
|
|
|
has constraintext => ( |
72
|
|
|
|
|
|
|
is => "rw", |
73
|
|
|
|
|
|
|
isa => enum( [ "inside", "outside", "both", "none" ] ), |
74
|
|
|
|
|
|
|
documentation => "Constrain the size of text inside or outside a bar to be no larger than the bar itself.", |
75
|
|
|
|
|
|
|
); |
76
|
|
|
|
|
|
|
|
77
|
|
|
|
|
|
|
has customdata => ( |
78
|
|
|
|
|
|
|
is => "rw", |
79
|
|
|
|
|
|
|
isa => "ArrayRef|PDL", |
80
|
|
|
|
|
|
|
documentation => |
81
|
|
|
|
|
|
|
"Assigns extra data each datum. This may be useful when listening to hover, click and selection events. Note that, *scatter* traces also appends customdata items in the markers DOM elements", |
82
|
|
|
|
|
|
|
); |
83
|
|
|
|
|
|
|
|
84
|
|
|
|
|
|
|
has customdatasrc => ( is => "rw", |
85
|
|
|
|
|
|
|
isa => "Str", |
86
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for customdata .", |
87
|
|
|
|
|
|
|
); |
88
|
|
|
|
|
|
|
|
89
|
|
|
|
|
|
|
has dx => ( is => "rw", |
90
|
|
|
|
|
|
|
isa => "Num", |
91
|
|
|
|
|
|
|
documentation => "Sets the x coordinate step. See `x0` for more info.", |
92
|
|
|
|
|
|
|
); |
93
|
|
|
|
|
|
|
|
94
|
|
|
|
|
|
|
has dy => ( is => "rw", |
95
|
|
|
|
|
|
|
isa => "Num", |
96
|
|
|
|
|
|
|
documentation => "Sets the y coordinate step. See `y0` for more info.", |
97
|
|
|
|
|
|
|
); |
98
|
|
|
|
|
|
|
|
99
|
|
|
|
|
|
|
has hoverinfo => ( |
100
|
|
|
|
|
|
|
is => "rw", |
101
|
|
|
|
|
|
|
isa => "Str|ArrayRef[Str]", |
102
|
|
|
|
|
|
|
documentation => |
103
|
|
|
|
|
|
|
"Determines which trace information appear on hover. If `none` or `skip` are set, no information is displayed upon hovering. But, if `none` is set, click and hover events are still fired.", |
104
|
|
|
|
|
|
|
); |
105
|
|
|
|
|
|
|
|
106
|
|
|
|
|
|
|
has hoverinfosrc => ( is => "rw", |
107
|
|
|
|
|
|
|
isa => "Str", |
108
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for hoverinfo .", |
109
|
|
|
|
|
|
|
); |
110
|
|
|
|
|
|
|
|
111
|
|
|
|
|
|
|
has hoverlabel => ( is => "rw", |
112
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Hoverlabel", ); |
113
|
|
|
|
|
|
|
|
114
|
|
|
|
|
|
|
has hovertemplate => ( |
115
|
|
|
|
|
|
|
is => "rw", |
116
|
|
|
|
|
|
|
isa => "Str|ArrayRef[Str]", |
117
|
|
|
|
|
|
|
documentation => |
118
|
|
|
|
|
|
|
"Template string used for rendering the information that appear on hover box. Note that this will override `hoverinfo`. Variables are inserted using %{variable}, for example \"y: %{y}\". Numbers are formatted using d3-format's syntax %{variable:d3-format}, for example \"Price: %{y:\$.2f}\". https://github.com/d3/d3-3.x-api-reference/blob/master/Formatting.md#d3_format for details on the formatting syntax. Dates are formatted using d3-time-format's syntax %{variable|d3-time-format}, for example \"Day: %{2019-01-01|%A}\". https://github.com/d3/d3-3.x-api-reference/blob/master/Time-Formatting.md#format for details on the date formatting syntax. The variables available in `hovertemplate` are the ones emitted as event data described at this link https://plot.ly/javascript/plotlyjs-events/#event-data. Additionally, every attributes that can be specified per-point (the ones that are `arrayOk: true`) are available. variables `percentInitial`, `percentPrevious` and `percentTotal`. Anything contained in tag `<extra>` is displayed in the secondary box, for example \"<extra>{fullData.name}</extra>\". To hide the secondary box completely, use an empty tag `<extra></extra>`.", |
119
|
|
|
|
|
|
|
); |
120
|
|
|
|
|
|
|
|
121
|
|
|
|
|
|
|
has hovertemplatesrc => ( is => "rw", |
122
|
|
|
|
|
|
|
isa => "Str", |
123
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for hovertemplate .", |
124
|
|
|
|
|
|
|
); |
125
|
|
|
|
|
|
|
|
126
|
|
|
|
|
|
|
has hovertext => ( |
127
|
|
|
|
|
|
|
is => "rw", |
128
|
|
|
|
|
|
|
isa => "Str|ArrayRef[Str]", |
129
|
|
|
|
|
|
|
documentation => |
130
|
|
|
|
|
|
|
"Sets hover text elements associated with each (x,y) pair. If a single string, the same string appears over all the data points. If an array of string, the items are mapped in order to the this trace's (x,y) coordinates. To be seen, trace `hoverinfo` must contain a *text* flag.", |
131
|
|
|
|
|
|
|
); |
132
|
|
|
|
|
|
|
|
133
|
|
|
|
|
|
|
has hovertextsrc => ( is => "rw", |
134
|
|
|
|
|
|
|
isa => "Str", |
135
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for hovertext .", |
136
|
|
|
|
|
|
|
); |
137
|
|
|
|
|
|
|
|
138
|
|
|
|
|
|
|
has ids => ( |
139
|
|
|
|
|
|
|
is => "rw", |
140
|
|
|
|
|
|
|
isa => "ArrayRef|PDL", |
141
|
|
|
|
|
|
|
documentation => |
142
|
|
|
|
|
|
|
"Assigns id labels to each datum. These ids for object constancy of data points during animation. Should be an array of strings, not numbers or any other type.", |
143
|
|
|
|
|
|
|
); |
144
|
|
|
|
|
|
|
|
145
|
|
|
|
|
|
|
has idssrc => ( is => "rw", |
146
|
|
|
|
|
|
|
isa => "Str", |
147
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for ids .", |
148
|
|
|
|
|
|
|
); |
149
|
|
|
|
|
|
|
|
150
|
|
|
|
|
|
|
has insidetextanchor => ( |
151
|
|
|
|
|
|
|
is => "rw", |
152
|
|
|
|
|
|
|
isa => enum( [ "end", "middle", "start" ] ), |
153
|
|
|
|
|
|
|
documentation => "Determines if texts are kept at center or start/end points in `textposition` *inside* mode.", |
154
|
|
|
|
|
|
|
); |
155
|
|
|
|
|
|
|
|
156
|
|
|
|
|
|
|
has insidetextfont => ( is => "rw", |
157
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Insidetextfont", ); |
158
|
|
|
|
|
|
|
|
159
|
|
|
|
|
|
|
has legendgroup => ( |
160
|
|
|
|
|
|
|
is => "rw", |
161
|
|
|
|
|
|
|
isa => "Str", |
162
|
|
|
|
|
|
|
documentation => |
163
|
|
|
|
|
|
|
"Sets the legend group for this trace. Traces part of the same legend group hide/show at the same time when toggling legend items.", |
164
|
|
|
|
|
|
|
); |
165
|
|
|
|
|
|
|
|
166
|
|
|
|
|
|
|
has marker => ( is => "rw", |
167
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Marker", ); |
168
|
|
|
|
|
|
|
|
169
|
|
|
|
|
|
|
has pmeta => ( |
170
|
|
|
|
|
|
|
is => "rw", |
171
|
|
|
|
|
|
|
isa => "Any|ArrayRef[Any]", |
172
|
|
|
|
|
|
|
documentation => |
173
|
|
|
|
|
|
|
"Assigns extra meta information associated with this trace that can be used in various text attributes. Attributes such as trace `name`, graph, axis and colorbar `title.text`, annotation `text` `rangeselector`, `updatemenues` and `sliders` `label` text all support `meta`. To access the trace `meta` values in an attribute in the same trace, simply use `%{meta[i]}` where `i` is the index or key of the `meta` item in question. To access trace `meta` in layout attributes, use `%{data[n[.meta[i]}` where `i` is the index or key of the `meta` and `n` is the trace index.", |
174
|
|
|
|
|
|
|
); |
175
|
|
|
|
|
|
|
|
176
|
|
|
|
|
|
|
has metasrc => ( is => "rw", |
177
|
|
|
|
|
|
|
isa => "Str", |
178
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for meta .", |
179
|
|
|
|
|
|
|
); |
180
|
|
|
|
|
|
|
|
181
|
|
|
|
|
|
|
has name => ( is => "rw", |
182
|
|
|
|
|
|
|
isa => "Str", |
183
|
|
|
|
|
|
|
documentation => "Sets the trace name. The trace name appear as the legend item and on hover.", |
184
|
|
|
|
|
|
|
); |
185
|
|
|
|
|
|
|
|
186
|
|
|
|
|
|
|
has offset => ( |
187
|
|
|
|
|
|
|
is => "rw", |
188
|
|
|
|
|
|
|
isa => "Num", |
189
|
|
|
|
|
|
|
documentation => |
190
|
|
|
|
|
|
|
"Shifts the position where the bar is drawn (in position axis units). In *group* barmode, traces that set *offset* will be excluded and drawn in *overlay* mode instead.", |
191
|
|
|
|
|
|
|
); |
192
|
|
|
|
|
|
|
|
193
|
|
|
|
|
|
|
has offsetgroup => ( |
194
|
|
|
|
|
|
|
is => "rw", |
195
|
|
|
|
|
|
|
isa => "Str", |
196
|
|
|
|
|
|
|
documentation => |
197
|
|
|
|
|
|
|
"Set several traces linked to the same position axis or matching axes to the same offsetgroup where bars of the same position coordinate will line up.", |
198
|
|
|
|
|
|
|
); |
199
|
|
|
|
|
|
|
|
200
|
|
|
|
|
|
|
has opacity => ( is => "rw", |
201
|
|
|
|
|
|
|
isa => "Num", |
202
|
|
|
|
|
|
|
documentation => "Sets the opacity of the trace.", |
203
|
|
|
|
|
|
|
); |
204
|
|
|
|
|
|
|
|
205
|
|
|
|
|
|
|
has orientation => ( |
206
|
|
|
|
|
|
|
is => "rw", |
207
|
|
|
|
|
|
|
isa => enum( [ "v", "h" ] ), |
208
|
|
|
|
|
|
|
documentation => |
209
|
|
|
|
|
|
|
"Sets the orientation of the funnels. With *v* (*h*), the value of the each bar spans along the vertical (horizontal). By default funnels are tend to be oriented horizontally; unless only *y* array is presented or orientation is set to *v*. Also regarding graphs including only 'horizontal' funnels, *autorange* on the *y-axis* are set to *reversed*.", |
210
|
|
|
|
|
|
|
); |
211
|
|
|
|
|
|
|
|
212
|
|
|
|
|
|
|
has outsidetextfont => ( is => "rw", |
213
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Outsidetextfont", ); |
214
|
|
|
|
|
|
|
|
215
|
|
|
|
|
|
|
has selectedpoints => ( |
216
|
|
|
|
|
|
|
is => "rw", |
217
|
|
|
|
|
|
|
isa => "Any", |
218
|
|
|
|
|
|
|
documentation => |
219
|
|
|
|
|
|
|
"Array containing integer indices of selected points. Has an effect only for traces that support selections. Note that an empty array means an empty selection where the `unselected` are turned on for all points, whereas, any other non-array values means no selection all where the `selected` and `unselected` styles have no effect.", |
220
|
|
|
|
|
|
|
); |
221
|
|
|
|
|
|
|
|
222
|
|
|
|
|
|
|
has showlegend => ( |
223
|
|
|
|
|
|
|
is => "rw", |
224
|
|
|
|
|
|
|
isa => "Bool", |
225
|
|
|
|
|
|
|
documentation => "Determines whether or not an item corresponding to this trace is shown in the legend.", |
226
|
|
|
|
|
|
|
); |
227
|
|
|
|
|
|
|
|
228
|
|
|
|
|
|
|
has stream => ( is => "rw", |
229
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Stream", ); |
230
|
|
|
|
|
|
|
|
231
|
|
|
|
|
|
|
has text => ( |
232
|
|
|
|
|
|
|
is => "rw", |
233
|
|
|
|
|
|
|
isa => "Str|ArrayRef[Str]", |
234
|
|
|
|
|
|
|
documentation => |
235
|
|
|
|
|
|
|
"Sets text elements associated with each (x,y) pair. If a single string, the same string appears over all the data points. If an array of string, the items are mapped in order to the this trace's (x,y) coordinates. If trace `hoverinfo` contains a *text* flag and *hovertext* is not set, these elements will be seen in the hover labels.", |
236
|
|
|
|
|
|
|
); |
237
|
|
|
|
|
|
|
|
238
|
|
|
|
|
|
|
has textangle => ( |
239
|
|
|
|
|
|
|
is => "rw", |
240
|
|
|
|
|
|
|
documentation => |
241
|
|
|
|
|
|
|
"Sets the angle of the tick labels with respect to the bar. For example, a `tickangle` of -90 draws the tick labels vertically. With *auto* the texts may automatically be rotated to fit with the maximum size in bars.", |
242
|
|
|
|
|
|
|
); |
243
|
|
|
|
|
|
|
|
244
|
|
|
|
|
|
|
has textfont => ( is => "rw", |
245
|
|
|
|
|
|
|
isa => "Maybe[HashRef]|Chart::Plotly::Trace::Funnel::Textfont", ); |
246
|
|
|
|
|
|
|
|
247
|
|
|
|
|
|
|
has textinfo => ( |
248
|
|
|
|
|
|
|
is => "rw", |
249
|
|
|
|
|
|
|
isa => "Str", |
250
|
|
|
|
|
|
|
documentation => |
251
|
|
|
|
|
|
|
"Determines which trace information appear on the graph. In the case of having multiple funnels, percentages & totals are computed separately (per trace).", |
252
|
|
|
|
|
|
|
); |
253
|
|
|
|
|
|
|
|
254
|
|
|
|
|
|
|
has textposition => ( |
255
|
|
|
|
|
|
|
is => "rw", |
256
|
|
|
|
|
|
|
isa => union( [ enum( [ "inside", "outside", "auto", "none" ] ), "ArrayRef" ] ), |
257
|
|
|
|
|
|
|
documentation => |
258
|
|
|
|
|
|
|
"Specifies the location of the `text`. *inside* positions `text` inside, next to the bar end (rotated and scaled if needed). *outside* positions `text` outside, next to the bar end (scaled if needed), unless there is another bar stacked on this one, then the text gets pushed inside. *auto* tries to position `text` inside the bar, but if the bar is too small and no bar is stacked on this one the text is moved outside.", |
259
|
|
|
|
|
|
|
); |
260
|
|
|
|
|
|
|
|
261
|
|
|
|
|
|
|
has textpositionsrc => ( is => "rw", |
262
|
|
|
|
|
|
|
isa => "Str", |
263
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for textposition .", |
264
|
|
|
|
|
|
|
); |
265
|
|
|
|
|
|
|
|
266
|
|
|
|
|
|
|
has textsrc => ( is => "rw", |
267
|
|
|
|
|
|
|
isa => "Str", |
268
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for text .", |
269
|
|
|
|
|
|
|
); |
270
|
|
|
|
|
|
|
|
271
|
|
|
|
|
|
|
has texttemplate => ( |
272
|
|
|
|
|
|
|
is => "rw", |
273
|
|
|
|
|
|
|
isa => "Str|ArrayRef[Str]", |
274
|
|
|
|
|
|
|
documentation => |
275
|
|
|
|
|
|
|
"Template string used for rendering the information text that appear on points. Note that this will override `textinfo`. Variables are inserted using %{variable}, for example \"y: %{y}\". Numbers are formatted using d3-format's syntax %{variable:d3-format}, for example \"Price: %{y:\$.2f}\". https://github.com/d3/d3-3.x-api-reference/blob/master/Formatting.md#d3_format for details on the formatting syntax. Dates are formatted using d3-time-format's syntax %{variable|d3-time-format}, for example \"Day: %{2019-01-01|%A}\". https://github.com/d3/d3-3.x-api-reference/blob/master/Time-Formatting.md#format for details on the date formatting syntax. Every attributes that can be specified per-point (the ones that are `arrayOk: true`) are available. variables `percentInitial`, `percentPrevious`, `percentTotal`, `label` and `value`.", |
276
|
|
|
|
|
|
|
); |
277
|
|
|
|
|
|
|
|
278
|
|
|
|
|
|
|
has texttemplatesrc => ( is => "rw", |
279
|
|
|
|
|
|
|
isa => "Str", |
280
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for texttemplate .", |
281
|
|
|
|
|
|
|
); |
282
|
|
|
|
|
|
|
|
283
|
|
|
|
|
|
|
has transforms => ( is => "rw", |
284
|
|
|
|
|
|
|
isa => "ArrayRef|ArrayRef[Chart::Plotly::Trace::Funnel::Transform]", ); |
285
|
|
|
|
|
|
|
|
286
|
|
|
|
|
|
|
has uid => ( |
287
|
|
|
|
|
|
|
is => "rw", |
288
|
|
|
|
|
|
|
isa => "Str", |
289
|
|
|
|
|
|
|
documentation => |
290
|
|
|
|
|
|
|
"Assign an id to this trace, Use this to provide object constancy between traces during animations and transitions.", |
291
|
|
|
|
|
|
|
); |
292
|
|
|
|
|
|
|
|
293
|
|
|
|
|
|
|
has uirevision => ( |
294
|
|
|
|
|
|
|
is => "rw", |
295
|
|
|
|
|
|
|
isa => "Any", |
296
|
|
|
|
|
|
|
documentation => |
297
|
|
|
|
|
|
|
"Controls persistence of some user-driven changes to the trace: `constraintrange` in `parcoords` traces, as well as some `editable: true` modifications such as `name` and `colorbar.title`. Defaults to `layout.uirevision`. Note that other user-driven trace attribute changes are controlled by `layout` attributes: `trace.visible` is controlled by `layout.legend.uirevision`, `selectedpoints` is controlled by `layout.selectionrevision`, and `colorbar.(x|y)` (accessible with `config: {editable: true}`) is controlled by `layout.editrevision`. Trace changes are tracked by `uid`, which only falls back on trace index if no `uid` is provided. So if your app can add/remove traces before the end of the `data` array, such that the same trace has a different index, you can still preserve user-driven changes if you give each trace a `uid` that stays with it as it moves.", |
298
|
|
|
|
|
|
|
); |
299
|
|
|
|
|
|
|
|
300
|
|
|
|
|
|
|
has visible => ( |
301
|
|
|
|
|
|
|
is => "rw", |
302
|
|
|
|
|
|
|
documentation => |
303
|
|
|
|
|
|
|
"Determines whether or not this trace is visible. If *legendonly*, the trace is not drawn, but can appear as a legend item (provided that the legend itself is visible).", |
304
|
|
|
|
|
|
|
); |
305
|
|
|
|
|
|
|
|
306
|
|
|
|
|
|
|
has width => ( is => "rw", |
307
|
|
|
|
|
|
|
isa => "Num", |
308
|
|
|
|
|
|
|
documentation => "Sets the bar width (in position axis units).", |
309
|
|
|
|
|
|
|
); |
310
|
|
|
|
|
|
|
|
311
|
|
|
|
|
|
|
has x => ( is => "rw", |
312
|
|
|
|
|
|
|
isa => "ArrayRef|PDL", |
313
|
|
|
|
|
|
|
documentation => "Sets the x coordinates.", |
314
|
|
|
|
|
|
|
); |
315
|
|
|
|
|
|
|
|
316
|
|
|
|
|
|
|
has x0 => ( |
317
|
|
|
|
|
|
|
is => "rw", |
318
|
|
|
|
|
|
|
isa => "Any", |
319
|
|
|
|
|
|
|
documentation => |
320
|
|
|
|
|
|
|
"Alternate to `x`. Builds a linear space of x coordinates. Use with `dx` where `x0` is the starting coordinate and `dx` the step.", |
321
|
|
|
|
|
|
|
); |
322
|
|
|
|
|
|
|
|
323
|
|
|
|
|
|
|
has xaxis => ( |
324
|
|
|
|
|
|
|
is => "rw", |
325
|
|
|
|
|
|
|
documentation => |
326
|
|
|
|
|
|
|
"Sets a reference between this trace's x coordinates and a 2D cartesian x axis. If *x* (the default value), the x coordinates refer to `layout.xaxis`. If *x2*, the x coordinates refer to `layout.xaxis2`, and so on.", |
327
|
|
|
|
|
|
|
); |
328
|
|
|
|
|
|
|
|
329
|
|
|
|
|
|
|
has xsrc => ( is => "rw", |
330
|
|
|
|
|
|
|
isa => "Str", |
331
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for x .", |
332
|
|
|
|
|
|
|
); |
333
|
|
|
|
|
|
|
|
334
|
|
|
|
|
|
|
has y => ( is => "rw", |
335
|
|
|
|
|
|
|
isa => "ArrayRef|PDL", |
336
|
|
|
|
|
|
|
documentation => "Sets the y coordinates.", |
337
|
|
|
|
|
|
|
); |
338
|
|
|
|
|
|
|
|
339
|
|
|
|
|
|
|
has y0 => ( |
340
|
|
|
|
|
|
|
is => "rw", |
341
|
|
|
|
|
|
|
isa => "Any", |
342
|
|
|
|
|
|
|
documentation => |
343
|
|
|
|
|
|
|
"Alternate to `y`. Builds a linear space of y coordinates. Use with `dy` where `y0` is the starting coordinate and `dy` the step.", |
344
|
|
|
|
|
|
|
); |
345
|
|
|
|
|
|
|
|
346
|
|
|
|
|
|
|
has yaxis => ( |
347
|
|
|
|
|
|
|
is => "rw", |
348
|
|
|
|
|
|
|
documentation => |
349
|
|
|
|
|
|
|
"Sets a reference between this trace's y coordinates and a 2D cartesian y axis. If *y* (the default value), the y coordinates refer to `layout.yaxis`. If *y2*, the y coordinates refer to `layout.yaxis2`, and so on.", |
350
|
|
|
|
|
|
|
); |
351
|
|
|
|
|
|
|
|
352
|
|
|
|
|
|
|
has ysrc => ( is => "rw", |
353
|
|
|
|
|
|
|
isa => "Str", |
354
|
|
|
|
|
|
|
documentation => "Sets the source reference on plot.ly for y .", |
355
|
|
|
|
|
|
|
); |
356
|
|
|
|
|
|
|
|
357
|
|
|
|
|
|
|
__PACKAGE__->meta->make_immutable(); |
358
|
|
|
|
|
|
|
1; |
359
|
|
|
|
|
|
|
|
360
|
|
|
|
|
|
|
__END__ |
361
|
|
|
|
|
|
|
|
362
|
|
|
|
|
|
|
=pod |
363
|
|
|
|
|
|
|
|
364
|
|
|
|
|
|
|
=encoding utf-8 |
365
|
|
|
|
|
|
|
|
366
|
|
|
|
|
|
|
=head1 NAME |
367
|
|
|
|
|
|
|
|
368
|
|
|
|
|
|
|
Chart::Plotly::Trace::Funnel - Visualize stages in a process using length-encoded bars. This trace can be used to show data in either a part-to-whole representation wherein each item appears in a single stage, or in a "drop-off" representation wherein each item appears in each stage it traversed. See also the "funnelarea" trace type for a different approach to visualizing funnel data. |
369
|
|
|
|
|
|
|
|
370
|
|
|
|
|
|
|
=head1 VERSION |
371
|
|
|
|
|
|
|
|
372
|
|
|
|
|
|
|
version 0.041 |
373
|
|
|
|
|
|
|
|
374
|
|
|
|
|
|
|
=head1 SYNOPSIS |
375
|
|
|
|
|
|
|
|
376
|
|
|
|
|
|
|
use Chart::Plotly; |
377
|
|
|
|
|
|
|
use Chart::Plotly::Plot; |
378
|
|
|
|
|
|
|
use JSON; |
379
|
|
|
|
|
|
|
use Chart::Plotly::Trace::Funnel; |
380
|
|
|
|
|
|
|
|
381
|
|
|
|
|
|
|
# Example from https://github.com/plotly/plotly.js/blob/b93e3a5a83b6561ac6258a59f274b5fc87630c3e/test/image/mocks/funnel_11.json |
382
|
|
|
|
|
|
|
my $trace1 = Chart::Plotly::Trace::Funnel->new({'orientation' => 'v', 'marker' => {'color' => 'rgb(255, 102, 97)', }, 'y' => [13.23, 22.7, 26.06, ], 'x' => ['Half Dose', 'Full Dose', 'Double Dose', ], 'name' => 'Orange Juice', }); |
383
|
|
|
|
|
|
|
|
384
|
|
|
|
|
|
|
my $trace2 = Chart::Plotly::Trace::Funnel->new({'name' => 'Vitamin C', 'marker' => {'color' => 'rgb(0, 196, 200)', }, 'y' => [7.98, 16.77, 26.14, ], 'x' => ['Half Dose', 'Full Dose', 'Double Dose', ], 'orientation' => 'v', }); |
385
|
|
|
|
|
|
|
|
386
|
|
|
|
|
|
|
my $trace3 = Chart::Plotly::Trace::Funnel->new({'name' => 'Std Dev - OJ', 'x' => ['Half Dose', 'Full Dose', 'Double Dose', ], 'y' => [1.4102837, 1.236752, 0.8396031, ], 'visible' => JSON::false, 'orientation' => 'v', }); |
387
|
|
|
|
|
|
|
|
388
|
|
|
|
|
|
|
my $trace4 = Chart::Plotly::Trace::Funnel->new({'y' => [0.868562, 0.7954104, 1.5171757, ], 'x' => ['Half Dose', 'Full Dose', 'Double Dose', ], 'name' => 'Std Dev - VC', 'orientation' => 'v', 'visible' => JSON::false, }); |
389
|
|
|
|
|
|
|
|
390
|
|
|
|
|
|
|
|
391
|
|
|
|
|
|
|
my $plot = Chart::Plotly::Plot->new( |
392
|
|
|
|
|
|
|
traces => [$trace1, $trace2, $trace3, $trace4, ], |
393
|
|
|
|
|
|
|
layout => |
394
|
|
|
|
|
|
|
{'autosize' => JSON::false, 'hidesources' => JSON::false, 'plot_bgcolor' => 'rgb(217, 217, 217)', 'font' => {'color' => '#000', 'size' => 12, 'family' => 'Arial, sans-serif', }, 'width' => 600, 'separators' => '.,', 'legend' => {'xanchor' => 'left', 'font' => {'size' => 16, 'family' => '', 'color' => 'rgb(0, 0, 0)', }, 'bgcolor' => 'rgba(255, 255, 255, 0)', 'bordercolor' => 'rgba(0, 0, 0, 0)', 'yanchor' => 'auto', 'x' => 1.02, 'y' => 0.931907250442406, 'borderwidth' => 1, 'traceorder' => 'normal', }, 'funnelgroupgap' => 0, 'funnelgap' => 0.2, 'annotations' => [{'tag' => '', 'yatype' => 'linear', 'showarrow' => JSON::false, 'xanchor' => 'auto', 'bgcolor' => 'rgba(0,0,0,0)', 'arrowhead' => 1, 'yref' => 'paper', 'ax' => -10, 'align' => 'center', 'yanchor' => 'auto', 'xatype' => 'category', 'bordercolor' => '', 'ref' => 'paper', 'text' => '<b>Supplement</b>', 'x' => 1.3479735318445, 'y' => 0.998214285714286, 'opacity' => 1, 'arrowwidth' => 0, 'font' => {'size' => 18, 'family' => '', 'color' => '', }, 'ay' => -26.7109375, 'arrowcolor' => '', 'borderpad' => 1, 'borderwidth' => 1, 'xref' => 'paper', 'arrowsize' => 1, }, ], 'height' => 440, 'dragmode' => 'zoom', 'hovermode' => 'x', 'paper_bgcolor' => '#fff', 'boxmode' => 'overlay', 'showlegend' => JSON::true, 'titlefont' => {'family' => '', 'size' => 16, 'color' => '', }, 'title' => 'Grouped Funnel Chart', 'margin' => {'r' => 0, 't' => 80, 'b' => 80, 'l' => 80, 'autoexpand' => JSON::true, 'pad' => 2, }, 'yaxis' => {'gridcolor' => 'rgb(255, 255, 255)', 'autotick' => JSON::true, 'ticks' => '', 'tickfont' => {'color' => '', 'size' => 16, 'family' => '', }, 'mirror' => JSON::true, 'tickangle' => 0, 'domain' => [0, 1, ], 'anchor' => 'x', 'showgrid' => JSON::true, 'exponentformat' => 'e', 'tick0' => 0, 'showticklabels' => JSON::true, 'nticks' => 0, 'zerolinewidth' => 1, 'zeroline' => JSON::false, 'position' => 0, 'range' => [0, 29.1128165263158, ], 'dtick' => 5, 'showexponent' => 'all', 'showline' => JSON::false, 'linecolor' => '#000', 'type' => 'linear', 'linewidth' => 0.1, 'overlaying' => JSON::false, 'ticklen' => 5, 'rangemode' => 'normal', 'tickwidth' => 1, 'tickcolor' => '#000', 'title' => 'Length', 'autorange' => JSON::true, 'titlefont' => {'size' => 16, 'family' => '', 'color' => '', }, 'zerolinecolor' => '#000', 'gridwidth' => 1.9, }, 'funnelmode' => 'group', 'xaxis' => {'ticks' => '', 'autotick' => JSON::true, 'gridcolor' => 'rgb(255, 255, 255)', 'tickfont' => {'color' => '', 'family' => '', 'size' => 16, }, 'mirror' => JSON::true, 'anchor' => 'y', 'tickangle' => 0, 'domain' => [0, 1, ], 'showgrid' => JSON::true, 'exponentformat' => 'e', 'tick0' => 0, 'showticklabels' => JSON::true, 'range' => [-0.5, 2.5, ], 'nticks' => 0, 'zerolinewidth' => 1, 'zeroline' => JSON::false, 'position' => 0, 'showexponent' => 'all', 'dtick' => 1, 'showline' => JSON::false, 'type' => 'category', 'linecolor' => '#000', 'overlaying' => JSON::false, 'linewidth' => 0.1, 'ticklen' => 5, 'rangemode' => 'normal', 'titlefont' => {'size' => 16, 'family' => '', 'color' => '', }, 'autorange' => JSON::true, 'title' => 'Dose (mg)', 'tickwidth' => 1, 'tickcolor' => '#000', 'gridwidth' => 1.9, 'zerolinecolor' => '#000', }, } |
395
|
|
|
|
|
|
|
); |
396
|
|
|
|
|
|
|
|
397
|
|
|
|
|
|
|
Chart::Plotly::show_plot($plot); |
398
|
|
|
|
|
|
|
|
399
|
|
|
|
|
|
|
=head1 DESCRIPTION |
400
|
|
|
|
|
|
|
|
401
|
|
|
|
|
|
|
Visualize stages in a process using length-encoded bars. This trace can be used to show data in either a part-to-whole representation wherein each item appears in a single stage, or in a "drop-off" representation wherein each item appears in each stage it traversed. See also the "funnelarea" trace type for a different approach to visualizing funnel data. |
402
|
|
|
|
|
|
|
|
403
|
|
|
|
|
|
|
Screenshot of the above example: |
404
|
|
|
|
|
|
|
|
405
|
|
|
|
|
|
|
=for HTML <p> |
406
|
|
|
|
|
|
|
<img src="https://raw.githubusercontent.com/pablrod/p5-Chart-Plotly/master/examples/traces/funnel.png" alt="Screenshot of the above example"> |
407
|
|
|
|
|
|
|
</p> |
408
|
|
|
|
|
|
|
|
409
|
|
|
|
|
|
|
=for markdown 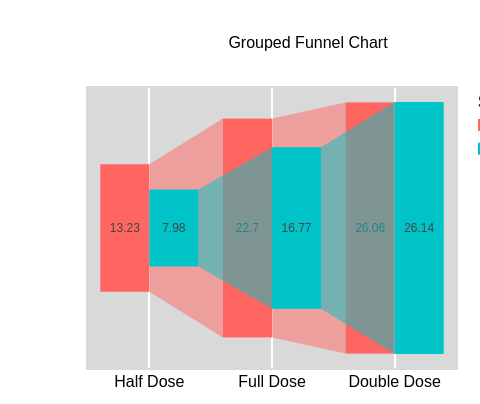 |
410
|
|
|
|
|
|
|
|
411
|
|
|
|
|
|
|
=for HTML <p> |
412
|
|
|
|
|
|
|
<iframe src="https://raw.githubusercontent.com/pablrod/p5-Chart-Plotly/master/examples/traces/funnel.html" style="border:none;" width="80%" height="520"></iframe> |
413
|
|
|
|
|
|
|
</p> |
414
|
|
|
|
|
|
|
|
415
|
|
|
|
|
|
|
This file has been autogenerated from the official plotly.js source. |
416
|
|
|
|
|
|
|
|
417
|
|
|
|
|
|
|
If you like Plotly, please support them: L<https://plot.ly/> |
418
|
|
|
|
|
|
|
Open source announcement: L<https://plot.ly/javascript/open-source-announcement/> |
419
|
|
|
|
|
|
|
|
420
|
|
|
|
|
|
|
Full reference: L<https://plot.ly/javascript/reference/#funnel> |
421
|
|
|
|
|
|
|
|
422
|
|
|
|
|
|
|
=head1 DISCLAIMER |
423
|
|
|
|
|
|
|
|
424
|
|
|
|
|
|
|
This is an unofficial Plotly Perl module. Currently I'm not affiliated in any way with Plotly. |
425
|
|
|
|
|
|
|
But I think plotly.js is a great library and I want to use it with perl. |
426
|
|
|
|
|
|
|
|
427
|
|
|
|
|
|
|
=head1 METHODS |
428
|
|
|
|
|
|
|
|
429
|
|
|
|
|
|
|
=head2 TO_JSON |
430
|
|
|
|
|
|
|
|
431
|
|
|
|
|
|
|
Serialize the trace to JSON. This method should be called only by L<JSON> serializer. |
432
|
|
|
|
|
|
|
|
433
|
|
|
|
|
|
|
=head2 type |
434
|
|
|
|
|
|
|
|
435
|
|
|
|
|
|
|
Trace type. |
436
|
|
|
|
|
|
|
|
437
|
|
|
|
|
|
|
=head1 ATTRIBUTES |
438
|
|
|
|
|
|
|
|
439
|
|
|
|
|
|
|
=over |
440
|
|
|
|
|
|
|
|
441
|
|
|
|
|
|
|
=item * alignmentgroup |
442
|
|
|
|
|
|
|
|
443
|
|
|
|
|
|
|
Set several traces linked to the same position axis or matching axes to the same alignmentgroup. This controls whether bars compute their positional range dependently or independently. |
444
|
|
|
|
|
|
|
|
445
|
|
|
|
|
|
|
=item * cliponaxis |
446
|
|
|
|
|
|
|
|
447
|
|
|
|
|
|
|
Determines whether the text nodes are clipped about the subplot axes. To show the text nodes above axis lines and tick labels, make sure to set `xaxis.layer` and `yaxis.layer` to *below traces*. |
448
|
|
|
|
|
|
|
|
449
|
|
|
|
|
|
|
=item * connector |
450
|
|
|
|
|
|
|
|
451
|
|
|
|
|
|
|
=item * constraintext |
452
|
|
|
|
|
|
|
|
453
|
|
|
|
|
|
|
Constrain the size of text inside or outside a bar to be no larger than the bar itself. |
454
|
|
|
|
|
|
|
|
455
|
|
|
|
|
|
|
=item * customdata |
456
|
|
|
|
|
|
|
|
457
|
|
|
|
|
|
|
Assigns extra data each datum. This may be useful when listening to hover, click and selection events. Note that, *scatter* traces also appends customdata items in the markers DOM elements |
458
|
|
|
|
|
|
|
|
459
|
|
|
|
|
|
|
=item * customdatasrc |
460
|
|
|
|
|
|
|
|
461
|
|
|
|
|
|
|
Sets the source reference on plot.ly for customdata . |
462
|
|
|
|
|
|
|
|
463
|
|
|
|
|
|
|
=item * dx |
464
|
|
|
|
|
|
|
|
465
|
|
|
|
|
|
|
Sets the x coordinate step. See `x0` for more info. |
466
|
|
|
|
|
|
|
|
467
|
|
|
|
|
|
|
=item * dy |
468
|
|
|
|
|
|
|
|
469
|
|
|
|
|
|
|
Sets the y coordinate step. See `y0` for more info. |
470
|
|
|
|
|
|
|
|
471
|
|
|
|
|
|
|
=item * hoverinfo |
472
|
|
|
|
|
|
|
|
473
|
|
|
|
|
|
|
Determines which trace information appear on hover. If `none` or `skip` are set, no information is displayed upon hovering. But, if `none` is set, click and hover events are still fired. |
474
|
|
|
|
|
|
|
|
475
|
|
|
|
|
|
|
=item * hoverinfosrc |
476
|
|
|
|
|
|
|
|
477
|
|
|
|
|
|
|
Sets the source reference on plot.ly for hoverinfo . |
478
|
|
|
|
|
|
|
|
479
|
|
|
|
|
|
|
=item * hoverlabel |
480
|
|
|
|
|
|
|
|
481
|
|
|
|
|
|
|
=item * hovertemplate |
482
|
|
|
|
|
|
|
|
483
|
|
|
|
|
|
|
Template string used for rendering the information that appear on hover box. Note that this will override `hoverinfo`. Variables are inserted using %{variable}, for example "y: %{y}". Numbers are formatted using d3-format's syntax %{variable:d3-format}, for example "Price: %{y:$.2f}". https://github.com/d3/d3-3.x-api-reference/blob/master/Formatting.md#d3_format for details on the formatting syntax. Dates are formatted using d3-time-format's syntax %{variable|d3-time-format}, for example "Day: %{2019-01-01|%A}". https://github.com/d3/d3-3.x-api-reference/blob/master/Time-Formatting.md#format for details on the date formatting syntax. The variables available in `hovertemplate` are the ones emitted as event data described at this link https://plot.ly/javascript/plotlyjs-events/#event-data. Additionally, every attributes that can be specified per-point (the ones that are `arrayOk: true`) are available. variables `percentInitial`, `percentPrevious` and `percentTotal`. Anything contained in tag `<extra>` is displayed in the secondary box, for example "<extra>{fullData.name}</extra>". To hide the secondary box completely, use an empty tag `<extra></extra>`. |
484
|
|
|
|
|
|
|
|
485
|
|
|
|
|
|
|
=item * hovertemplatesrc |
486
|
|
|
|
|
|
|
|
487
|
|
|
|
|
|
|
Sets the source reference on plot.ly for hovertemplate . |
488
|
|
|
|
|
|
|
|
489
|
|
|
|
|
|
|
=item * hovertext |
490
|
|
|
|
|
|
|
|
491
|
|
|
|
|
|
|
Sets hover text elements associated with each (x,y) pair. If a single string, the same string appears over all the data points. If an array of string, the items are mapped in order to the this trace's (x,y) coordinates. To be seen, trace `hoverinfo` must contain a *text* flag. |
492
|
|
|
|
|
|
|
|
493
|
|
|
|
|
|
|
=item * hovertextsrc |
494
|
|
|
|
|
|
|
|
495
|
|
|
|
|
|
|
Sets the source reference on plot.ly for hovertext . |
496
|
|
|
|
|
|
|
|
497
|
|
|
|
|
|
|
=item * ids |
498
|
|
|
|
|
|
|
|
499
|
|
|
|
|
|
|
Assigns id labels to each datum. These ids for object constancy of data points during animation. Should be an array of strings, not numbers or any other type. |
500
|
|
|
|
|
|
|
|
501
|
|
|
|
|
|
|
=item * idssrc |
502
|
|
|
|
|
|
|
|
503
|
|
|
|
|
|
|
Sets the source reference on plot.ly for ids . |
504
|
|
|
|
|
|
|
|
505
|
|
|
|
|
|
|
=item * insidetextanchor |
506
|
|
|
|
|
|
|
|
507
|
|
|
|
|
|
|
Determines if texts are kept at center or start/end points in `textposition` *inside* mode. |
508
|
|
|
|
|
|
|
|
509
|
|
|
|
|
|
|
=item * insidetextfont |
510
|
|
|
|
|
|
|
|
511
|
|
|
|
|
|
|
=item * legendgroup |
512
|
|
|
|
|
|
|
|
513
|
|
|
|
|
|
|
Sets the legend group for this trace. Traces part of the same legend group hide/show at the same time when toggling legend items. |
514
|
|
|
|
|
|
|
|
515
|
|
|
|
|
|
|
=item * marker |
516
|
|
|
|
|
|
|
|
517
|
|
|
|
|
|
|
=item * pmeta |
518
|
|
|
|
|
|
|
|
519
|
|
|
|
|
|
|
Assigns extra meta information associated with this trace that can be used in various text attributes. Attributes such as trace `name`, graph, axis and colorbar `title.text`, annotation `text` `rangeselector`, `updatemenues` and `sliders` `label` text all support `meta`. To access the trace `meta` values in an attribute in the same trace, simply use `%{meta[i]}` where `i` is the index or key of the `meta` item in question. To access trace `meta` in layout attributes, use `%{data[n[.meta[i]}` where `i` is the index or key of the `meta` and `n` is the trace index. |
520
|
|
|
|
|
|
|
|
521
|
|
|
|
|
|
|
=item * metasrc |
522
|
|
|
|
|
|
|
|
523
|
|
|
|
|
|
|
Sets the source reference on plot.ly for meta . |
524
|
|
|
|
|
|
|
|
525
|
|
|
|
|
|
|
=item * name |
526
|
|
|
|
|
|
|
|
527
|
|
|
|
|
|
|
Sets the trace name. The trace name appear as the legend item and on hover. |
528
|
|
|
|
|
|
|
|
529
|
|
|
|
|
|
|
=item * offset |
530
|
|
|
|
|
|
|
|
531
|
|
|
|
|
|
|
Shifts the position where the bar is drawn (in position axis units). In *group* barmode, traces that set *offset* will be excluded and drawn in *overlay* mode instead. |
532
|
|
|
|
|
|
|
|
533
|
|
|
|
|
|
|
=item * offsetgroup |
534
|
|
|
|
|
|
|
|
535
|
|
|
|
|
|
|
Set several traces linked to the same position axis or matching axes to the same offsetgroup where bars of the same position coordinate will line up. |
536
|
|
|
|
|
|
|
|
537
|
|
|
|
|
|
|
=item * opacity |
538
|
|
|
|
|
|
|
|
539
|
|
|
|
|
|
|
Sets the opacity of the trace. |
540
|
|
|
|
|
|
|
|
541
|
|
|
|
|
|
|
=item * orientation |
542
|
|
|
|
|
|
|
|
543
|
|
|
|
|
|
|
Sets the orientation of the funnels. With *v* (*h*), the value of the each bar spans along the vertical (horizontal). By default funnels are tend to be oriented horizontally; unless only *y* array is presented or orientation is set to *v*. Also regarding graphs including only 'horizontal' funnels, *autorange* on the *y-axis* are set to *reversed*. |
544
|
|
|
|
|
|
|
|
545
|
|
|
|
|
|
|
=item * outsidetextfont |
546
|
|
|
|
|
|
|
|
547
|
|
|
|
|
|
|
=item * selectedpoints |
548
|
|
|
|
|
|
|
|
549
|
|
|
|
|
|
|
Array containing integer indices of selected points. Has an effect only for traces that support selections. Note that an empty array means an empty selection where the `unselected` are turned on for all points, whereas, any other non-array values means no selection all where the `selected` and `unselected` styles have no effect. |
550
|
|
|
|
|
|
|
|
551
|
|
|
|
|
|
|
=item * showlegend |
552
|
|
|
|
|
|
|
|
553
|
|
|
|
|
|
|
Determines whether or not an item corresponding to this trace is shown in the legend. |
554
|
|
|
|
|
|
|
|
555
|
|
|
|
|
|
|
=item * stream |
556
|
|
|
|
|
|
|
|
557
|
|
|
|
|
|
|
=item * text |
558
|
|
|
|
|
|
|
|
559
|
|
|
|
|
|
|
Sets text elements associated with each (x,y) pair. If a single string, the same string appears over all the data points. If an array of string, the items are mapped in order to the this trace's (x,y) coordinates. If trace `hoverinfo` contains a *text* flag and *hovertext* is not set, these elements will be seen in the hover labels. |
560
|
|
|
|
|
|
|
|
561
|
|
|
|
|
|
|
=item * textangle |
562
|
|
|
|
|
|
|
|
563
|
|
|
|
|
|
|
Sets the angle of the tick labels with respect to the bar. For example, a `tickangle` of -90 draws the tick labels vertically. With *auto* the texts may automatically be rotated to fit with the maximum size in bars. |
564
|
|
|
|
|
|
|
|
565
|
|
|
|
|
|
|
=item * textfont |
566
|
|
|
|
|
|
|
|
567
|
|
|
|
|
|
|
=item * textinfo |
568
|
|
|
|
|
|
|
|
569
|
|
|
|
|
|
|
Determines which trace information appear on the graph. In the case of having multiple funnels, percentages & totals are computed separately (per trace). |
570
|
|
|
|
|
|
|
|
571
|
|
|
|
|
|
|
=item * textposition |
572
|
|
|
|
|
|
|
|
573
|
|
|
|
|
|
|
Specifies the location of the `text`. *inside* positions `text` inside, next to the bar end (rotated and scaled if needed). *outside* positions `text` outside, next to the bar end (scaled if needed), unless there is another bar stacked on this one, then the text gets pushed inside. *auto* tries to position `text` inside the bar, but if the bar is too small and no bar is stacked on this one the text is moved outside. |
574
|
|
|
|
|
|
|
|
575
|
|
|
|
|
|
|
=item * textpositionsrc |
576
|
|
|
|
|
|
|
|
577
|
|
|
|
|
|
|
Sets the source reference on plot.ly for textposition . |
578
|
|
|
|
|
|
|
|
579
|
|
|
|
|
|
|
=item * textsrc |
580
|
|
|
|
|
|
|
|
581
|
|
|
|
|
|
|
Sets the source reference on plot.ly for text . |
582
|
|
|
|
|
|
|
|
583
|
|
|
|
|
|
|
=item * texttemplate |
584
|
|
|
|
|
|
|
|
585
|
|
|
|
|
|
|
Template string used for rendering the information text that appear on points. Note that this will override `textinfo`. Variables are inserted using %{variable}, for example "y: %{y}". Numbers are formatted using d3-format's syntax %{variable:d3-format}, for example "Price: %{y:$.2f}". https://github.com/d3/d3-3.x-api-reference/blob/master/Formatting.md#d3_format for details on the formatting syntax. Dates are formatted using d3-time-format's syntax %{variable|d3-time-format}, for example "Day: %{2019-01-01|%A}". https://github.com/d3/d3-3.x-api-reference/blob/master/Time-Formatting.md#format for details on the date formatting syntax. Every attributes that can be specified per-point (the ones that are `arrayOk: true`) are available. variables `percentInitial`, `percentPrevious`, `percentTotal`, `label` and `value`. |
586
|
|
|
|
|
|
|
|
587
|
|
|
|
|
|
|
=item * texttemplatesrc |
588
|
|
|
|
|
|
|
|
589
|
|
|
|
|
|
|
Sets the source reference on plot.ly for texttemplate . |
590
|
|
|
|
|
|
|
|
591
|
|
|
|
|
|
|
=item * transforms |
592
|
|
|
|
|
|
|
|
593
|
|
|
|
|
|
|
=item * uid |
594
|
|
|
|
|
|
|
|
595
|
|
|
|
|
|
|
Assign an id to this trace, Use this to provide object constancy between traces during animations and transitions. |
596
|
|
|
|
|
|
|
|
597
|
|
|
|
|
|
|
=item * uirevision |
598
|
|
|
|
|
|
|
|
599
|
|
|
|
|
|
|
Controls persistence of some user-driven changes to the trace: `constraintrange` in `parcoords` traces, as well as some `editable: true` modifications such as `name` and `colorbar.title`. Defaults to `layout.uirevision`. Note that other user-driven trace attribute changes are controlled by `layout` attributes: `trace.visible` is controlled by `layout.legend.uirevision`, `selectedpoints` is controlled by `layout.selectionrevision`, and `colorbar.(x|y)` (accessible with `config: {editable: true}`) is controlled by `layout.editrevision`. Trace changes are tracked by `uid`, which only falls back on trace index if no `uid` is provided. So if your app can add/remove traces before the end of the `data` array, such that the same trace has a different index, you can still preserve user-driven changes if you give each trace a `uid` that stays with it as it moves. |
600
|
|
|
|
|
|
|
|
601
|
|
|
|
|
|
|
=item * visible |
602
|
|
|
|
|
|
|
|
603
|
|
|
|
|
|
|
Determines whether or not this trace is visible. If *legendonly*, the trace is not drawn, but can appear as a legend item (provided that the legend itself is visible). |
604
|
|
|
|
|
|
|
|
605
|
|
|
|
|
|
|
=item * width |
606
|
|
|
|
|
|
|
|
607
|
|
|
|
|
|
|
Sets the bar width (in position axis units). |
608
|
|
|
|
|
|
|
|
609
|
|
|
|
|
|
|
=item * x |
610
|
|
|
|
|
|
|
|
611
|
|
|
|
|
|
|
Sets the x coordinates. |
612
|
|
|
|
|
|
|
|
613
|
|
|
|
|
|
|
=item * x0 |
614
|
|
|
|
|
|
|
|
615
|
|
|
|
|
|
|
Alternate to `x`. Builds a linear space of x coordinates. Use with `dx` where `x0` is the starting coordinate and `dx` the step. |
616
|
|
|
|
|
|
|
|
617
|
|
|
|
|
|
|
=item * xaxis |
618
|
|
|
|
|
|
|
|
619
|
|
|
|
|
|
|
Sets a reference between this trace's x coordinates and a 2D cartesian x axis. If *x* (the default value), the x coordinates refer to `layout.xaxis`. If *x2*, the x coordinates refer to `layout.xaxis2`, and so on. |
620
|
|
|
|
|
|
|
|
621
|
|
|
|
|
|
|
=item * xsrc |
622
|
|
|
|
|
|
|
|
623
|
|
|
|
|
|
|
Sets the source reference on plot.ly for x . |
624
|
|
|
|
|
|
|
|
625
|
|
|
|
|
|
|
=item * y |
626
|
|
|
|
|
|
|
|
627
|
|
|
|
|
|
|
Sets the y coordinates. |
628
|
|
|
|
|
|
|
|
629
|
|
|
|
|
|
|
=item * y0 |
630
|
|
|
|
|
|
|
|
631
|
|
|
|
|
|
|
Alternate to `y`. Builds a linear space of y coordinates. Use with `dy` where `y0` is the starting coordinate and `dy` the step. |
632
|
|
|
|
|
|
|
|
633
|
|
|
|
|
|
|
=item * yaxis |
634
|
|
|
|
|
|
|
|
635
|
|
|
|
|
|
|
Sets a reference between this trace's y coordinates and a 2D cartesian y axis. If *y* (the default value), the y coordinates refer to `layout.yaxis`. If *y2*, the y coordinates refer to `layout.yaxis2`, and so on. |
636
|
|
|
|
|
|
|
|
637
|
|
|
|
|
|
|
=item * ysrc |
638
|
|
|
|
|
|
|
|
639
|
|
|
|
|
|
|
Sets the source reference on plot.ly for y . |
640
|
|
|
|
|
|
|
|
641
|
|
|
|
|
|
|
=back |
642
|
|
|
|
|
|
|
|
643
|
|
|
|
|
|
|
=head1 AUTHOR |
644
|
|
|
|
|
|
|
|
645
|
|
|
|
|
|
|
Pablo Rodríguez González <pablo.rodriguez.gonzalez@gmail.com> |
646
|
|
|
|
|
|
|
|
647
|
|
|
|
|
|
|
=head1 COPYRIGHT AND LICENSE |
648
|
|
|
|
|
|
|
|
649
|
|
|
|
|
|
|
This software is Copyright (c) 2020 by Pablo Rodríguez González. |
650
|
|
|
|
|
|
|
|
651
|
|
|
|
|
|
|
This is free software, licensed under: |
652
|
|
|
|
|
|
|
|
653
|
|
|
|
|
|
|
The MIT (X11) License |
654
|
|
|
|
|
|
|
|
655
|
|
|
|
|
|
|
=cut |